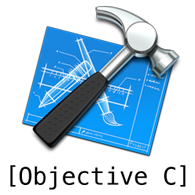
Programming in Objective-C
Overview
Objective-C has been Apple's language of choice for iOS App development since the iPhone first arrived on the scene in 2007. And with well over a million Apps in the App Store having been written in Objective-C, its importance and relevance remain crucial for any development team. This 3-day course will provide a thorough exploration of Object Oriented Programming with Objective-C through a myriad of hands-on labs and exercises that will cover both the theoretical and practical aspects of the language. Topics covered will include Objective-C Data Types, working with Collection Objects, Control-Flow, Creating Custom Classes, Inheritance, Polymorphism, Dynamic Binding and Dynamic Typing, the Foundation Framework, working with the iOS File System and much more.An in-depth exploration of Xcode - Apple's IDE for developing iOS Apps - will also be an integral part of this class as students will use it to write a myriad of programs that demonstrate the concepts being taught.
Students will have gained a solid foundation and understanding of how Objective-C works, a strong familiarity with the Xcode IDE, and thus be ideally prepared to move on to learning iOS App Development. Download Outline:
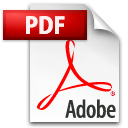
Course Objectives
- Learn to declare variables, write conditionals, loops, and use other building blocks of programming
- Understand how to work with Collection Objects such as NSArrays, NSDictionaries, and NSSets; use Fast Enumeration
- Create custom Classes, write Instance and Class Methods
- Understand Object-Oriented messaging, Inheritance, Polymorphism, Dynamic Binding and Dynamic Typing
- Learn to use the Foundation Framework's classes and data structures: NSString, NSNumber, NSDate, etc.
- Declare Structs and Enums
- Write full-fledged Objective-C Programs
Target Audience
Beginner programmers as well as experienced programmers who are not familiar with Objective-C.
Prerequisites
- Previous programming experience is recommended but not mandatory.
- Basic familiarity with Mac computers and working in Mac OS X is recommended
Lab Set-Up & Required Tools
- Mac computers running OS X 10.12 (“Sierra”) or greater. An iOS device is optional.
- Xcode 9 or greater(free download at: https://developer.apple.com/xcode)
- A Projector with mini-HDMI adapter for the Instructor
Course Outline
- Creating and Running an Objective-C Project in Xcode
- Xcode IDE overview
- Building and testing programs – the workflow
- Working with Data Types, Variables & Constants
- Declaring Variables, Performing Basic Operations
- Primitive Data Types: Ints, Floats, Doubles, Bools, Chars
- Strings – Literals, Mutability, Concatenation
- Logging variable values and string literals to Xcode's Console
- Type Casting
- Arithmetics and Expressions
- Addition, Subtraction, Multiplication, Division, Modulus
- Operator Precedence
- Assignment and Comparison operators
- Type conversions through mixed operations
- Introduction to the Math Library and its functions
- Control-Flow
- Conditionals
- IF and Ternary Statements
- Compound Relationals
- The Switch Statement
- Nested Conditionals
- Loops
- FOR loops, FOR-Condition-Increment
- WHILE loops, DO-WHILE loops
- Loop Control Statements: Continue, Break
- Conditionals
- Creating Custom Classes
- Declaring a custom class using @interface
- Implementing a custom class using @implementation
- Declaring Instance Variables and Instance Methods
- Creating Synthesized Accessor (Getter/Setter) Methods
- Instantiating Objects from Classes
- Sending Messages to Objects and Setting Object Properties
- Inheritance
- Base Classes, Creating Subclasses
- Overriding Methods & Properties
- Using Self
- Extension through Inheritance
- Polymorphism, Dynamic Typing and Dynamic Binding
- Same Method Names - Different Classes
- Static Typing
- Dynamic Typing and Dynamic Binding
- Run-time Querying of Objects and Classes
- Working with the ID Type
- The Foundation Framework and Collection Objects
- The NSNumber Class
- Working with NSString and NSMutableString
- Working with NSArrays and NSMutableArrays
- Working with NSDictionaries and NSMutableDictionaries
- Working with NSSet and NSMutableSet
- Fast Enumeration over Collection Objects
- Data Persistence and Working with iOS File System
- Working with the NSFileManager Class
- File-Paths and the NSURL Class
- Reading, writing and copying files.
- Working with Directories
- Working with NSData and NSMutableData
- Custom Data-Types, Protocols and Categories
- Declaring and working with Objective-C Structures
- Declaring and working with Objective-C Enums
- Renaming data-types with Typdef
- Extending Foundation Classes with Categories
- Understanding and creating Custom Protocols
- Archiving and Copying Objects
- Shallow vs. Deep Copying
- The NSCopying Protocol
- Archiving XML Property Lists
- Creating Encoding and Decoding Methods
- Working with NSKeyedArchiver
Note: Clients are welcomed to request customization of this course to meet any specific needs they may have. Additional lessons can be created and substituted for existing ones to create a custom class.